/// 欢迎数据 Model
class WelcomeModel {
/// 图片url
String? image;
/// 标题
String? title;
/// 说明
String? desc;
WelcomeModel({this.image, this.title, this.desc});
WelcomeModel.fromJson(dynamic json) {
image = json["image"];
title = json["title"];
desc = json["desc"];
}
Map<String, dynamic> toJson() {
var map = <String, dynamic>{};
map["image"] = image;
map["title"] = title;
map["desc"] = desc;
return map;
}
}
flutter_native_splash:
background_image_android: "assets/launcher/background.png"
background_image_ios: "assets/launcher/background.png"
android_12:
image: "assets/launcher/android12.png"
icon_background_color: "#324ea1"
# 图片填充样式
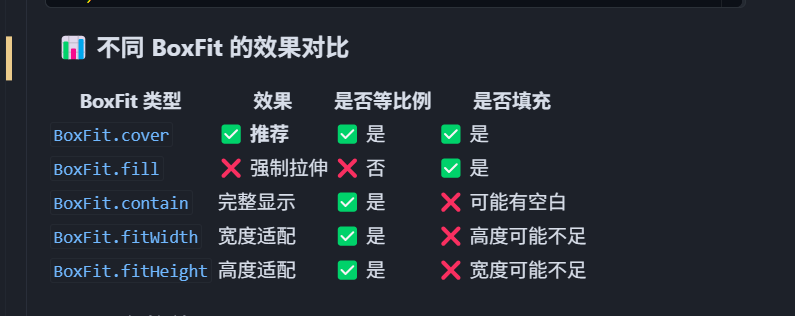
import 'package:flutter/material.dart';
import 'package:flutter_application_1/common/index.dart';
import 'package:get/get.dart';
import 'index.dart';
class SplashPage extends GetView<SplashController> {
const SplashPage({super.key});
// 主视图
Widget _buildView() {
return SizedBox.expand(
child: const ImageWidget.img(
AssetsImages.splash1Jpg,
fit: BoxFit.cover, // 等比例缩放,填充整个容器
),
);
}
@override
Widget build(BuildContext conte
import 'package:adaptive_theme/adaptive_theme.dart';
import 'package:af_ui_template/af_ui_template.dart';
import 'package:flutter/material.dart';
import 'package:flutter_application_1/common/index.dart';
import 'package:get/get_navigation/get_navigation.dart';
import 'global.dart';
void main() async {
await Global.init();
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
/**
* @param {number[]} nums - Array containing 3n integers
* @return {number} - Minimum difference between two parts after removing n elements
*/
var minimumDifference = function(nums) {
const n = nums.length / 3;
// Max heap for prefix sums (we want the smallest n elements for left side)
let maxHeap = [];
let leftSum = 0;
const leftDiff = new Array(n + 1);
// Initialize max heap and compute initial leftSum from first n elements
for (let i = 0; i < n; i++) {
https://fontdrop.info/
# снёс базу
bin/console aramuz:vitess:drop-schema --force
bin/console aramuz:vitess:init-schema
# накатил миграции
bin/console doctrine:migration:migrate --no-interaction
# обновил схему
bin/console aramuz:vitess:update-schema
# накати фикстуры
bin/console doctrine:fixture:load --no-interaction
add_action('woocommerce_order_status_completed', 'schedule_coupon_after_purchase');
function schedule_coupon_after_purchase($order_id)
{
$order = wc_get_order($order_id);
$user_email = $order->get_billing_email();
create_coupon_and_send_email($user_email);
}
add_action('create_delayed_coupon', 'create_coupon_and_send_email');
function create_coupon_and_send_email($user_email)
{
$coupon_code = 'thankyou-' . wp_generate_password(6, false); // generate unique code
$coupon
{% comment %}
Renders product-badges for product and card product
Accepts:
- product: {Object} product object.
- is_card: {Boolean} default false
Usage:
{%- render 'product-badges', product: product -%}
{% endcomment %}
{%- liquid
assign has_tags = false
assign wrapper_class = 'product__sticker'
assign badge_size = 'badge--lg'
if is_card == true
assign wrapper_class = 'card__badge'
assign badge_size = 'badge--md'
endif
if product.availabl
<?php
## Removing widgets from the WordPress Dashboard
add_action( 'wp_dashboard_setup', 'clear_wp_dash' );
function clear_wp_dash(){
$dash_side = & $GLOBALS['wp_meta_boxes']['dashboard']['side']['core'];
$dash_normal = & $GLOBALS['wp_meta_boxes']['dashboard']['normal']['core'];
unset( $dash_side['dashboard_quick_press'] ); // Quick publication
unset( $dash_side['dashboard_recent_drafts'] ); // Recent Drafts
unset( $dash_side['dashboard_primary'] ); // WordPress Blo
// debugging is the process of finding and
// fixing errors within a computer program
// errors in our C# programs are called exceptions
// exceptions are "thrown" when the program encounters an error
// let's create a simple program that throws an exception
int IntegerDivision(int x, int y)
{
return x / y;
}
// the program will throw an exception when we try to divide by zero
//int result = IntegerDivision(10, 0);
// exceptions are caught using try-catch blocks
// try-ca
// a return value is a value that is returned from a
// method to the code that called it
// methods with return values are called functions!
// a method can only have one return value
// and the return value must be of the same type
// as the method
// here is an example of a method with a return value
int Add(int a, int b)
{
return a + b;
}
// we can call the method like this
int sum = Add(5, 3);
// we can also call the method like this
int x = 5;
int y = 3;
int sum2
/*
void is a return type that means the method doesn't return any value
Key points about void:
No return value: The method performs an action but doesn't give back any data
No return statement: You don't need (and can't use) return with a value
Early exit: You can use return; (without a value) to exit the method early
Common use cases: Printing, updating variables, performing operations without needing to return results
/*
// a parameter is a variable in a method definition. When a m
// a foreach loop is used to iterate over a
// collection of values
// to be more specific, it iterates over
// things that are "IEnumerable"
// all collections are IEnumerable!
// here is what a foreach loop looks like
// foreach (Type thing in collectionOfThings)
// {
// // do something...
// }
// let's see a real example with a number array!
int[] numbers = { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
// what about with... a l
// while loops and do while loops are used
// to execute a block of code repeatedly.
// here is what a while loop looks like
// while (condition)
// {
// // code to execute
// }
// here is what a do while loop looks like
// do
// {
// // code to execute
// }
// while (condition)
// let's make some real ones!
// here is a while loop that counts to 5
int count = 0;
while (count < 5)
{
Console.WriteLine(count);
count++;
}
Console.WriteLine($"The total